Inserting in Excel file from C# collection using Open XML SDK 2.0
In this post I will show inserting rows in excel file from a c
Sharp list using Open XML SDK. Open XML SDK is very useful when you
don’t want to or cannot use Microsoft Office InterOP dll.
You can download it from below URL
http://www.microsoft.com/download/en/details.aspx?id=5124
Once you download and run the MSI follow the below steps.
Creating Data Source to be inserted in excel
Let us say you have class as below,
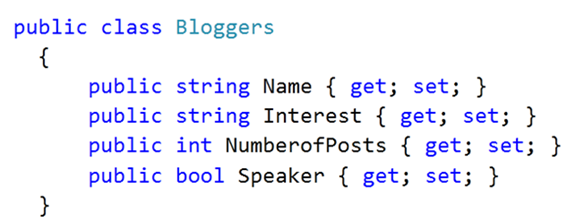
And below function returning list of bloggers. We are going to insert all the items from this list in Excel file.
01 | private List<Bloggers> GetDataToInsertInExcel() |
03 | List<Bloggers> lstBloggers = new List<Bloggers> |
08 | Interest = "SQL Server" , |
28 | Name = "Shiv Prasad Koirala" , |
35 | Name = "Anoop Madusudhan" , |
You are very much free to change data source to
- Azure table
- SQL Server table
- SQL Azure table
Theoretically you can use any data source provided you are converting
the result in List. If you are using SQL Server or SQL Azure, you can
use LINQ to SQL to create data source.
Since now we have data source, let us insert the items of list in the excel file using open xml SDK.
Add Namespaces
You need to add below namespaces,
Have a Template
If you notice we have four properties in entity class. So there would
be four columns in the excel sheet. Save an excel file with any name of
your preference at any location of your preference. For purpose of this
article I am saving it to the
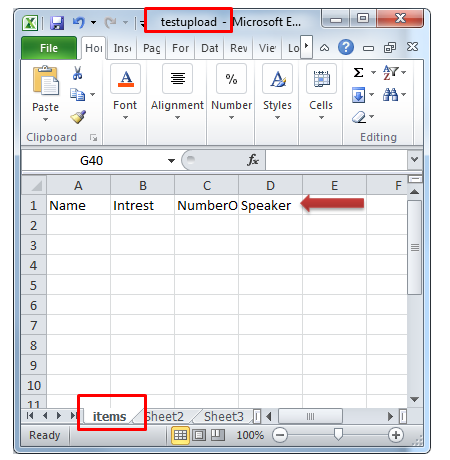
There are three points worth noticing about the template
- All the columns [properties of entity class] is in first row in columns A, B,C,D
- Sheet is renamed to items. If you want you can have default name.
- Template excel file with name testupload is in d drive.
Opening the template file to insert rows

If you have save template Excel file with different name in different
location then you will have to change the location in above code.
If you have changed the sheet name to
item then you will fetch it as below,
If you have not renamed the sheet and want to insert in the first
sheet, you can do like below. Make note of code in comment to fetch the
first sheet.
Inserting the rows
Now document is open, so we need to insert rows one by one. So we
will loop through all the items in list and call a function to create
row. On successful return of the row from function we will append it to
the open sheet.
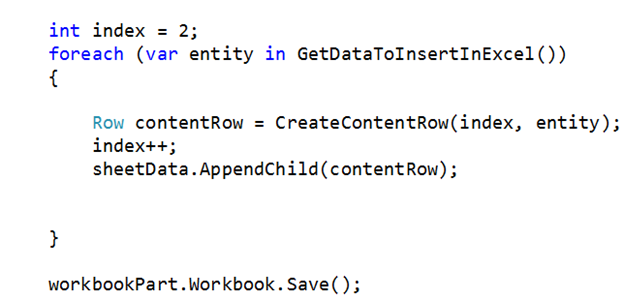
If you notice above code snippet I have initialized index value to 2
because in first row of the excel sheet, we are putting the header. From
second row onward items in each row would get inserted. I am making
call to
CreateContentRow function.
Creating the rows
In you notice above that
in header columns string array, we are starting from A to D. It is
because we have only four columns to insert. If you have 6 columns to
insert then string array would be from A to F.
In above snippet I am iterating through all the properties of the
entity object and creating cell reference by appending index with column
headers.
Next I need to find type of property .There may be three types
- String
- Integer
- Boolean
We need to check for the type of property and then create the cell to insert the value
Checking for String
Checking for Integer
Checking for Boolean
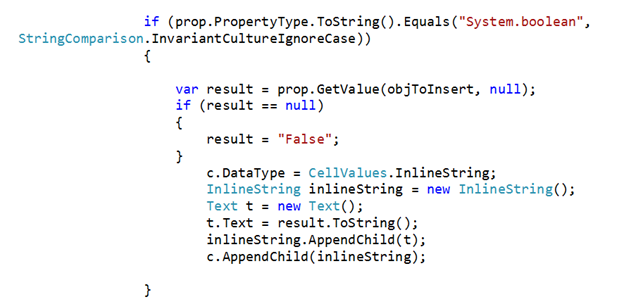
Putting all together all pieces of codes we discussed above, for your reference whole source code is as below,
002 | using System.Collections.Generic; |
003 | using DocumentFormat.OpenXml.Packaging; |
004 | using DocumentFormat.OpenXml.Spreadsheet; |
006 | namespace ConsoleApplication28 |
010 | static void Main( string [] args) |
012 | CreatingAndUploadingExcel(); |
014 | Public static bool CreatingAndUploadingExcel() |
017 | using (SpreadsheetDocument myWorkbook = SpreadsheetDocument.Open( "d:\\LocalCollection.xlsx" , true )) |
021 | WorkbookPart workbookPart = myWorkbook.WorkbookPart; |
023 | IEnumerable<Sheet>
Sheets =
myWorkbook.WorkbookPart.Workbook.GetFirstChild<Sheets>().Elements<Sheet>().Where(s=>s.Name== "items" ); |
024 | if (Sheets.Count() == 0) |
030 | string relationshipId = Sheets.First().Id.Value; |
031 | WorksheetPart worksheetPart = (WorksheetPart)myWorkbook.WorkbookPart.GetPartById(relationshipId); |
032 | SheetData sheetData = worksheetPart.Worksheet.GetFirstChild<SheetData>(); |
035 | foreach (var entity in GetDataToInsertInExcel()) |
038 | Row contentRow = CreateContentRow(index, entity); |
040 | sheetData.AppendChild(contentRow); |
043 | workbookPart.Workbook.Save(); |
048 | string [] headerColumns = new string [] { "A" , "B" , "C" , "D" }; |
049 | private Row CreateContentRow( int index, Bloggers objToInsert) |
053 | r.RowIndex = (UInt32) index; |
056 | foreach (var prop in objToInsert.GetType().GetProperties()) |
059 | c.CellReference = headerColumns[i].ToString() + index; |
061 | if (prop.PropertyType.ToString().Equals( "System.string" , StringComparison.InvariantCultureIgnoreCase)) |
064 | var result = prop.GetValue(objToInsert, null ); |
071 | c.DataType = CellValues.String; |
072 | InlineString inlineString = new InlineString(); |
074 | t.Text = result.ToString(); |
075 | inlineString.AppendChild(t); |
076 | c.AppendChild(inlineString); |
080 | if (prop.PropertyType.ToString().Equals( "System.int32" , StringComparison.InvariantCultureIgnoreCase)) |
083 | var result = prop.GetValue(objToInsert, null ); |
089 | CellValue v = new CellValue(); |
090 | v.Text = result.ToString(); |
095 | if (prop.PropertyType.ToString().Equals( "System.boolean" , StringComparison.InvariantCultureIgnoreCase)) |
098 | var result = prop.GetValue(objToInsert, null ); |
103 | c.DataType = CellValues.InlineString; |
104 | InlineString inlineString = new InlineString(); |
106 | t.Text = result.ToString(); |
107 | inlineString.AppendChild(t); |
108 | c.AppendChild(inlineString); |
120 | private List<Bloggers> GetDataToInsertInExcel() |
122 | List<Bloggers> lstBloggers = new List<Bloggers> |
127 | Interest = "SQL Server" , |
133 | Name = "Mahesh Chand" , |
147 | Name = "Shiv Prasad Koirala" , |
154 | Name = "Anoop Madusudhan" , |
169 | public string Name { get ; set ; } |
170 | public string Interest { get ; set ; } |
171 | public int NumberofPosts { get ; set ; } |
172 | public bool Speaker { get ; set ; } |
Now go ahead and open Excel file and you should get the row inserted. I hope this post was useful. Thanks for reading
Posted by
Dhananjay Kumar ⋅ October 4, 2011

No comments :
Post a Comment